Note: The examples mentioned in this article have source code available here.
PHP is not meant for desktop graphics programming. It just isn’t. PHP is web development language, or as you’ve seen in the PHP Dark Arts series, it can be used for some non-web related purposes. But using PHP for GUI development just isn’t something people do, so naturally I had to give it a shot. After much Google-fu I cam across the PHP-GTK project.
According to the PHP-GTK project site:
PHP-GTK is an extension for the PHP programming language that implements language bindings for GTK+. It provides an object-oriented interface to GTK+ classes and functions and greatly simplifies writing client-side cross-platform GUI applications
What Can You Do With It?
The first question that I had when I started looking at PHP-GTK was “What can I do with this?”. Simple GUIs are very possible with PHP-GTK. For example, you could easily build a questionnaire, a calculator, some sort of text editor, maybe a music library manager. In short, you can build simple desktop applications.
Where To Get PHP-GTK
Getting PHP-GTK is easy. Making it work for you is another matter. I had originally hoped to get it running on my Ubuntu 9.10 virtual machine that I do all of my Re-CycledAir development work on, but that just wasn’t working for me. I kept running into dependency issues and it just wouldn’t compile right. After much pain and suffering I decided to just use the pre-compiled Windows binary which worked like a charm. If you plan to follow any of these tutorials, I highly recommend that you use the Windows binary.
Once you have the Windows binary installed, just execute your GUI PHP programs using <path/to/phpgtk/>php.exe your_file.php.
Hello World
When I learn something new, be it a language or a library, I always like to start off with a simple “Hello World” program. What follows is a simple “Hello World” program that creates a small window and displays some text.
set_title('Hello Re-CycledAir'); //Tell GTK to quit the main loop when we close the window. This //is what allows the program to exit fully. $window->connect_simple('destroy', array('gtk', 'main_quit')); //Create a simple label that displays "Hello Re-CycledAir!" and then //add it to the window. $labelHello = new GtkLabel("Hello Re-CycledAir!"); $window->add($labelHello); //Make this window visible. $window->show_all(); //Start the main loop. Gtk::main(); ?> |
If everything goes correctly, you should get something that looks like this.
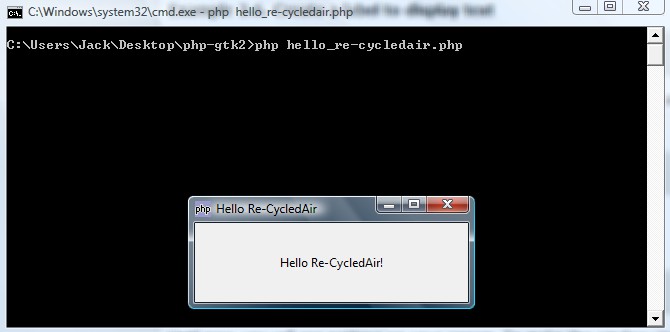
Hello World 2
In this example, we place a button on this window instead of a label, which then triggers a modal pop-up window with a message.
//Check to see if PHP-GTK has been loaded correctly. if (!class_exists('gtk')) { die("PHP-GTK has not been loaded in your php.ini file."); } //Create a new window and set it's title to "Hello Re-CycledAir". $window = new GtkWindow(); $window->set_title('Hello Re-CycledAir'); //Tell GTK to quit the main loop when we close the window. This //is what allows the program to exit fully. $window->connect_simple('destroy', array('gtk', 'main_quit')); //Add an OK button, connect it to the ok function, and //then add it to the window. $buttonOK = new GtkButton("_OK"); $buttonOK->connect_simple('clicked', 'ok', $window); $window->add($buttonOK); //Make this window visible. $window->show_all(); //Start the main loop. Gtk::main(); function ok(GtkWindow $window) { $message = "Hello again!"; //Create message dialog. $dialog = new GtkMessageDialog($window, Gtk::DIALOG_MODAL, Gtk::MESSAGE_ERROR, Gtk::BUTTONS_OK, $message); $dialog->set_markup( "You have received this message: \r\n" . "<span>" . $message . "</span>" ); //Run the dialog $dialog->run(); $dialog->destroy(); //Destroy the original window. $window->destroy(); } ?> |
If all goes well with this example, you should get output that looks like this:
Conlcusions
I am by no means a good GUI programmer. In fact, the only GUI programming I have done outside of these examples is building a few simple interfaces using Swing in Java. Your mileage may vary, but I found PHP-GTK to be a bit clumsy in how it handles things. Perhaps with some more development time it could become a better library, but generally there are much better languages and libraries out there for GUI development.
Note: The examples mentioned in this article have source code available here.
12 replies on “PHP Dark Arts: GUI Programming with GTK”
[…] This post was mentioned on Twitter by pok rap, Top Technology News and Hacker News, Re-CycledAir. Re-CycledAir said: PHP Dark Arts: GUI Programming with GTK http://fwds.me/4l […]
you source code have some “>” symbols, it is not beautiful to read, could you check it?
Thank you for making a comment about that. It turns out that the WP-Syntax plugin wasn’t properly decoding special characters. I hacked it a little bit to make it work, so there shouldn’t be anymore ugly non-decoded HTML characters in the code examples.
Once again, thanks!
[…] PHP Dark Arts: GUI Programming with GTK (re-cycledair.wploadtest.xyz) […]
[…] Gtk (tags: webdev php gtk) window.fbAsyncInit = function() { FB.init({appId: "", status: true, cookie: true, xfbml: true}); }; (function() { var e = document.createElement("script"); e.async = true; e.src = document.location.protocol + "//connect.facebook.net/en_US/all.js"; document.getElementById("fb-root").appendChild(e); }()); […]
[…] original here: PHP Dark Arts: GUI Programming with GTK | Re-Cycled Air 分类: php 标签: gtk, gui, php 评论 (0) Trackbacks (0) 发表评论 […]
Nah. I’m not sure. It’s nice to have it, but hardly anyone is using php-gtk. I guess it’s because PHP doesn’t lend itself to writing GUI apps. PHP5 got a few new Java syntax features, but is overall still a substandard OOP language. Python has less syntax clutches (e.g. private methods/properties) and thus comes way more natural at interfacing with Gtk.
However, for simple dialogs and minimal windows it might be a good idea. (But personally I think a FLTK binding would have been the better API choice for PHP.)
[…] Przeczytaj artykuł: PHP Dark Arts: GUI Programming with GTK | Re-Cycled Air […]
I think PHP GTK is nice to play around a bit, but please tell me, what could be the reason behind choosing to use PHP for desktop applications? I do almost all things i need programming wise with PHP, but im using my browser or the console as UI even on my local machine, and im quite happy with it. If i need to code apps like this i would rather not use PHP tbh.
Nevertheless its a nice Projekt even tho no real use for it, at least as far as i can imagine.
greets
Sandro
I couldn’t agree more. PHP is NOT a desktop programming language. This is exactly this post was part of the “Dark Arts” series, because it’s not something that you would normally use PHP for.
[…] PHP Gui Programming with GTK – Auch wenn man es nicht tun sollte, ist es nett zu lesen das es geht und wie es geht. Desktop Applikationen mit PHP […]
[…] Posted on October 10, 2010 […]